Recent Posts
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- Spring Batch
- 달인막창
- 깡돼후
- preemption #
- python
- PersistenceContext
- JanusWebRTCGateway
- kotlin
- Value too long for column
- k8s #kubernetes #쿠버네티스
- VARCHAR (1)
- PytestPluginManager
- 겨울 부산
- 코루틴 컨텍스트
- pytest
- tolerated
- mp4fpsmod
- 헥사고날아키텍처 #육각형아키텍처 #유스케이스
- JanusWebRTC
- 티스토리챌린지
- JanusGateway
- JanusWebRTCServer
- 코루틴 빌더
- 오블완
- table not found
- taint
- 자원부족
- terminal
- 개성국밥
- vfr video
Archives
너와 나의 스토리
모두를 위한 딥러닝 - RNN 실습 (5) 본문
반응형
모두를 위한 딥러닝 ML lab12-6: RNN with Time Series Data 강의 정리 & 코드
전체 코드: https://github.com/hunkim/DeepLearningZeroToAll/blob/master/lab-12-5-rnn_stock_prediction.py
RNN으로 time series data인 주식 시장을 예측해보자
Many to one
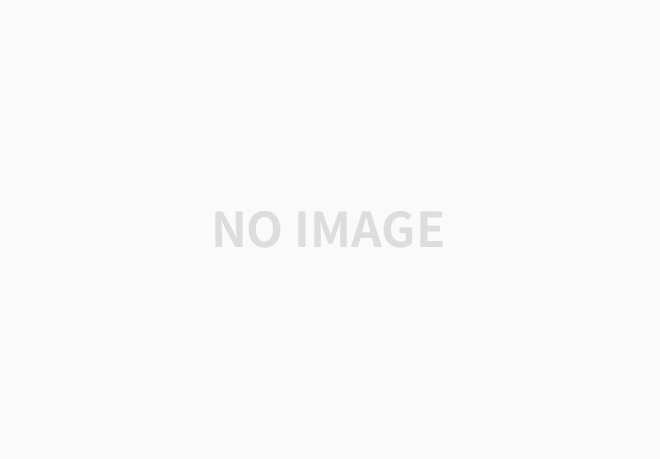
Dataset
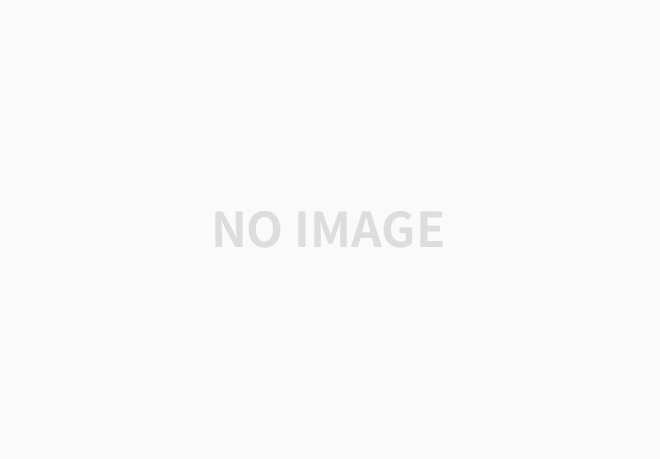
input dimension = 5 [open, high, low, volume, close]
sequence = 7 [7일 동안의 데이터를 토대로 수행]
output dimension = 1 [8일 째만 예측]
1. import
import tensorflow as tf
import numpy as np
import matplotlib
import os
# 그래프 수준의 난수 시드 설정
tf.set_random_seed(777)
if "DISPLAY" not in os.environ:
matplotlib.use('Agg')
import matplotlib.pyplot as plt
2. MinMaxScaler 함수
값이 매우 큰 값도 있고, 작은 값도 있기 때문에 MinMaxScaler함수로 normalize해준다
# parameters-data: normalized된 input data
# return-data : nomalized된 데이터, shape: [batch size, dimension]
def MinMaxScaler(data):
#분자
numerator = data - np.min(data,0)
#분모
denominator = np.max(data,0) -np.min(data,0)
#noise term prevents the zero division
return numerator / (denominator+1e-7)
3. sequence 및 dimension 선택
timesteps = seq_length =7
data_dim = 5
output_dim =1
hidden_dim=10
learning_rate =0.01
iterations =500
hidden_dim은 우리가 알아서 적당히 해주면 됨
4. 데이터 로드 및 정렬
# Open, High, Low, Volume, Close
xy = np.loadtxt('./input/data-02-stock_daily.csv', delimiter=',')
xy = xy[::-1] # reverse order (chronically ordered)
#ㄴ시간순으로 만들기 위해서 뒤집어 줌
#값이 들쑥날쑥함. (값이 큰 값도 있고 작은 값도 있음) -> MinMaxScaler()로 normalize
xy=MinMaxScaler(xy)
x=xy # x는 전체를 가짐
y= xy[:, [-1]] #y는 close 레이블된 값만 가짐
* xy[ :, [-1] ] 작동 예시
a=np.array([[1,2,3],[4,5,6],[7,8,9]])
a=a[:,[-1]]
a
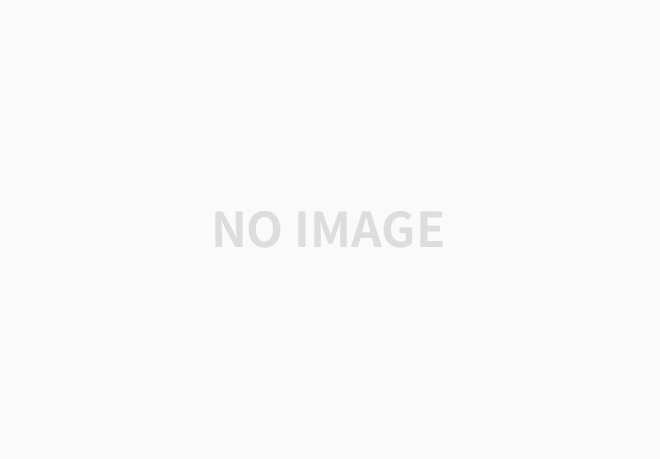
5. x, y 지정
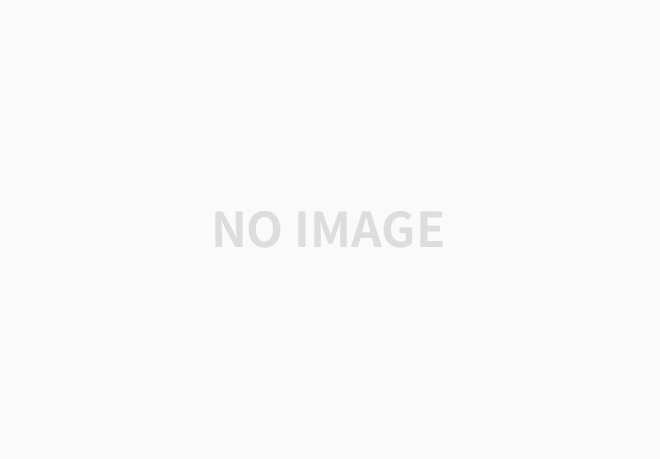
0~6을 x라고 하면 7번째 값이 y가 됨
dataX=[]
dataY=[]
for i in range(0, len(y)-seq_length):
_x=x[i:i+seq_length]
_y=y[i+seq_length]
print(_x,"->",_y)
dataX.append(_x)
dataY.append(_y)
6. dataset을 Training과 test로 나누기
# split to train and testing
train_size = int(len(dataY)*0.7)
test_size = len(dataY)-train_size
trainX, testX = np.array(dataX[0:train_size]),np.array(dataX[train_size:len(dataX)])
trainY, testY = np.array(dataY[0:train_size]),np.array(dataY[train_size:len(dataY)])
#input placeholders
#X는 sequence data
X= tf.placeholder(tf.float32, [None, seq_length, data_dim]) #[배치 사이즈, ,]
Y = tf.placeholder(tf.float32, [None,1]) # 출력 값이 한 개
7. LSTM and Loss
#LSTM and Loss
cell = tf.contrib.rnn.BasicLSTMCell(num_units=hidden_dim, state_is_tuple=True)
outputs, _states = tf.nn.dynamic_rnn(cell,X,dtype=tf.float32)
# 예측한 것에 fully_connected한 것을 실제 얘측으로 봄
# [:,-1] output의 마지막 값만 쓴다
# output_dim = 1
Y_pred = tf.contrib.layers.fully_connected(outputs[:,-1],output_dim, activation_fn=None)
# cost/loss
loss = tf.reduce_sum(tf.square(Y_pred-Y)) # sum of the squares
# optimizer
optimizer = tf.train.AdamOptimizer(0.01)
train=optimizer.minimize(loss)
8. Training and Results
#Training and Results
sess=tf.Session()
sess.run(tf.global_variables_initializer())
for i in range(1000):
_, l =sess.run([train,loss], feed_dict={X:trainX,Y:trainY})
print(i,l)
testPredict = sess.run(Y_pred, feed_dict={X:testX})
plt.plot(testY)
plt.plot(testPredict)
plt.show()
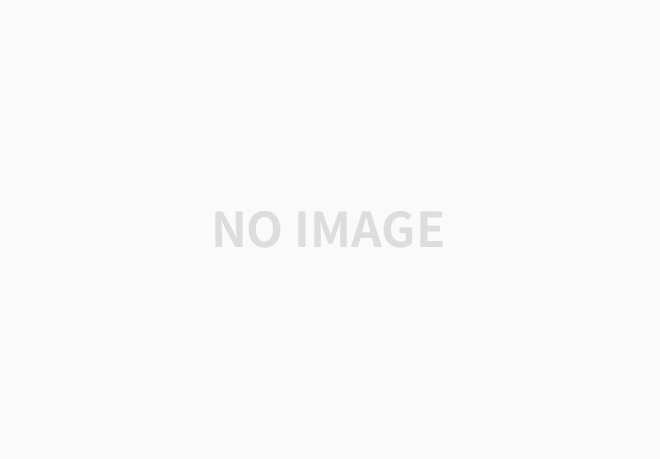
반응형
'Data Analysis > Machine learning' 카테고리의 다른 글
Training - BPTT / RTRL / EKF (0) | 2019.08.13 |
---|---|
SGD / EKF / PF algorithm (0) | 2019.08.12 |
"Efficient Online Learning Algorithms Based on LSTM Neural Networks" 논문 소개 & 개념 정리 - LSTM 기반의 online learning (0) | 2019.08.10 |
Deep learning - ANN / CNN (0) | 2019.08.09 |
모두를 위한 딥러닝 - RNN 실습(4) (0) | 2019.08.07 |